Golang Map
Understanding Maps in Go - A Powerful Data Structure for Key-Value Pairing
4/9/20232 min read
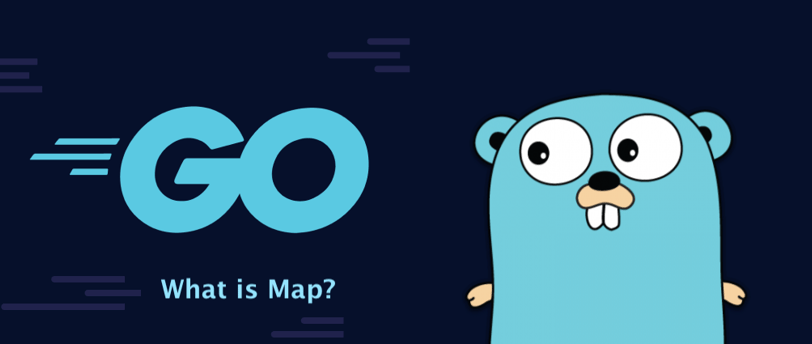
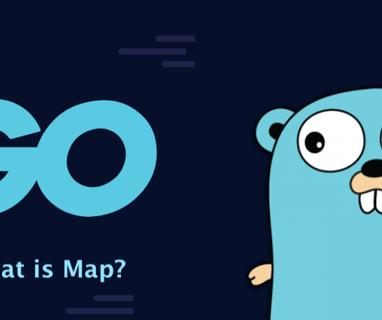
If you're familiar with Go (also known as Golang), you've likely come across maps, which are an essential data structure in the language. Maps provide a powerful way to store and retrieve values using keys, making them ideal for tasks such as counting occurrences of items, caching results, or building lookup tables.
In this blog post, we'll dive into the details of maps in Go and explore their various features and capabilities. We'll cover how to declare, initialize, access, modify, and delete key-value pairs in a map. We'll also discuss how to check for the existence of a key in a map and iterate over its key-value pairs. Additionally, we'll explore some best practices for using maps efficiently and effectively in your Go programs.
With code examples and practical explanations, this blog post aims to provide a comprehensive understanding of maps in Go, including their syntax, behavior, and common use cases. Whether you're a beginner learning Go or an experienced Go developer looking to sharpen your skills, this blog post will serve as a valuable reference for mastering the use of maps in your Go programs.
Topics Covered:
Introduction to maps in Go
Declaring and initializing maps
Accessing and modifying map values
Adding and deleting key-value pairs in a map
Checking for key existence in a map
Iterating over key-value pairs in a map
Best practices for using maps in Go
By the end of this blog post, you'll have a solid understanding of maps in Go and be able to leverage this powerful data structure in your own Go projects to store and retrieve data in an efficient and organized manner.
In this example, we declare and initialize a map ages with string keys and integer values. We then demonstrate how to access, modify, add, and delete key-value pairs in the map. We also show how to check if a key exists in the map using the optional second return value when accessing a map key. Finally, we iterate over the key-value pairs in the map using a for loop and the range keyword.
In Go (Golang), a map is a built-in data structure that provides an unordered collection of key-value pairs. Maps are also known as hash maps, dictionaries, or associative arrays in other programming languages. Here's an example of how to use a map in Go: